ADC DAC interfacing with FPGA | ADC DAC VHDL code
This page describes ADC DAC interfacing with FPGA. The ADC VHDL Code is used to read data from ADC to receive. The DAC VHDL code is used to write data to DAC for transmit.
Introduction:
As shown in the figure-1, 12 bit ADC and 14 bit DAC are interfaced with FPGA.
FPGA uses 16 I/O pins to interface ADC/DAC to have parallel and fast read/write access.
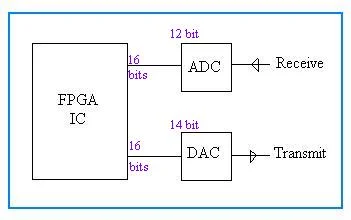
ADC converts analog signal to digital data format. The ADC VHDL code for receiver part is mentioned below in which 12 bits are converted to 16 bits with four leading zeroes for FPGA to read the digital data from ADC.
ADC DAC Interface Pin Diagram

The figure-2 depcits ADC DAC interface pin diagram for both receive and transmit section. DAC VHDL code for transmit side can also be coded similar to ADC VHDL code mentioned below with slight modifications.
ADC VHDL Code
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_ARITH.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity adc_dac_interface is port
(
CLK_SAMP : in std_logic;
reset : in std_logic;
enable_in : in std_logic;
Real_in_from_ADC : in std_logic_vector(11 downto 0);--receiver side i/p
imag_in_from_ADC : in std_logic_vector(11 downto 0); --receiver side i/p
Real_adc_to_phy : out std_logic_vector(15 downto 0);--receiver side 0/p
imag_adc_to_phy : out std_logic_vector(15 downto 0);--receiver side o/p
ADC_CLK1 : out std_logic;
ADC_CLK2 : out std_logic;
enable_out:out std_logic
);
end entity;
architecture beh of adc_dac_interface is
begin
process(CLK_SAMP,reset)
begin
if reset='1' then
enable_out<='0';
real_adc_to_phy<=(others=>'0');
imag_adc_to_phy<=(others=>'0');
elsif CLK_SAMP='1' and CLK_SAMP'event then
real_adc_to_phy<=Real_in_from_ADC (11)&Real_in_from_ADC (11)&Real_in_from_ADC (11)&Real_in_from_ADC (11)&Real_in_from_ADC ;
imag_adc_to_phy<= imag_in_from_ADC(11)& imag_in_from_ADC(11)& imag_in_from_ADC(11)& imag_in_from_ADC(11)& imag_in_from_ADC;
enable_out<=enable_in;
end if;
end process;
ADC_CLK1<=CLK_SAMP;
ADC_CLK2<=CLK_SAMP;
end beh;
ADC DAC Serial data interfacing with FPGA
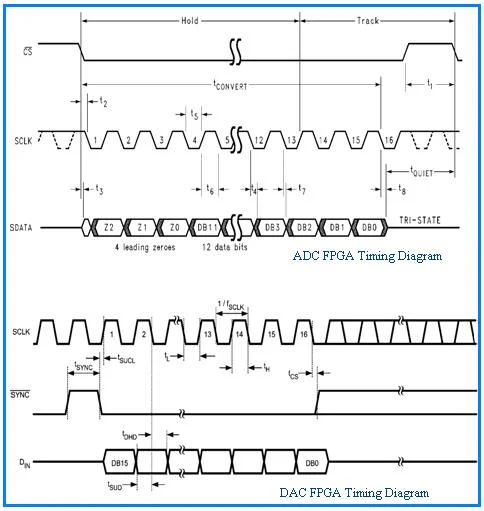
The figure-3 depicts ADC and DAC serial data interface timing diagram obtained from datasheets of the ADC/DAC. Both ADC and DAC are 12 bit analog to digital converter and 12 bit digital to analog converters respectively.
In ADC FPGA interface, CS is pulled low and then four zeroes are being transmitted which is followed by 12 bits. The 12 bit represents voltage acquired by the ADC. MSB is the first to be clocked. ADC FPGA VHDL/VERILOG code for the same can be easily written.
In DAC FPGA interface, SYNC is pulled HIGH and then 16 bits are being written. First 2 bits are zeroes, next 2 bits are configuration bits and rest 12 bits are actual information to be converted to analog format for transmission. DAC FPGA VHDL/VERILOG code for the same can be easily written.
USEFUL LINKS to VHDL CODES
Refer following as well as links mentioned on left side panel for useful VHDL codes.
D Flipflop
T Flipflop
Read Write RAM
4X1 MUX
4 bit binary counter
Radix4 Butterfly
16QAM Modulation
2bit Parallel to serial
RF and Wireless tutorials
WLAN 802.11ac 802.11ad wimax Zigbee z-wave GSM LTE UMTS Bluetooth UWB IoT satellite Antenna RADAR